Freemail API
Sample
Freemail API
API Documentation
Learn how to integrate Freemail API into your applications
Getting Started
Everything you need to know to start using the Freemail API
Authentication
All API requests require authentication using your API key. You can find your API key in the API Keys section of your dashboard.
Base URL
https://freemail.maev.rocks/send
Rate Limits
The free tier allows up to 300 emails per month. Rate limiting is applied to prevent abuse.
Sending Emails
Learn how to send emails using the API
Endpoint
POST /send
Request Parameters
import requests
url = "https://freemail.maev.rocks/send"
payload = {
"api_key": "your_api_key",
"sender_name": "Your Name",
"subject": "Hello from Freemail API",
"message": "This is a test email sent using Freemail API.",
"message_type": "plain",
"receiver_email": "recipient@example.com"
}
response = requests.post(url, json=payload)
print(response.json())
curl -X POST https://freemail.maev.rocks/send \
-H "Content-Type: application/json" \
-d '{
"api_key": "your_api_key",
"sender_name": "Your Name",
"subject": "Hello from Freemail API",
"message": "This is a test email sent using Freemail API.",
"message_type": "plain",
"receiver_email": "recipient@example.com"
}'
// Using fetch
const sendEmail = async () => {
const response = await fetch('https://freemail.maev.rocks/send', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
api_key: 'your_api_key',
sender_name: 'Your Name',
subject: 'Hello from Freemail API',
message: 'This is a test email sent using Freemail API.',
message_type: 'plain',
receiver_email: 'recipient@example.com'
})
});
const data = await response.json();
console.log(data);
};
sendEmail();
package main
import (
"bytes"
"encoding/json"
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://freemail.maev.rocks/send"
data := map[string]string{
"api_key": "your_api_key",
"sender_name": "Your Name",
"subject": "Hello from Freemail API",
"message": "This is a test email sent using Freemail API.",
"message_type": "plain",
"receiver_email": "recipient@example.com",
}
jsonData, _ := json.Marshal(data)
resp, err := http.Post(url, "application/json", bytes.NewBuffer(jsonData))
if err != nil {
fmt.Println("Error:", err)
return
}
defer resp.Body.Close()
body, _ := ioutil.ReadAll(resp.Body)
fmt.Println("Response:", string(body))
}
use reqwest::Client;
use serde_json::json;
use tokio;
#[tokio::main]
async fn main() -> Result<(), reqwest::Error> {
let client = Client::new();
let url = "https://freemail.maev.rocks/send";
let payload = json!({
"api_key": "your_api_key",
"sender_name": "Your Name",
"subject": "Hello from Freemail API",
"message": "This is a test email sent using Freemail API.",
"message_type": "plain",
"receiver_email": "recipient@example.com"
});
let response = client
.post(url)
.header("Content-Type", "application/json")
.json(&payload)
.send()
.await?;
println!("Response: {:?}", response.text().await?);
Ok(())
}
Response
{
"status": "success",
"message": "Email sent successfully",
"email_id": "email_12345"
}
Error Responses
{
"status": "error",
"message": "Invalid API key"
}
{
"status": "error",
"message": "Missing required parameters"
}
{
"status": "error",
"message": "Rate limit exceeded"
}
Retrieving Email Information
Learn how to retrieve details about sent emails
Endpoint
POST /email_info
Request Parameters
import requests
url = "https://freemail.maev.rocks/send/email_info"
payload = {
"email_id": "12345",
"api_key": "your_api_key"
}
response = requests.post(url, json=payload)
print(response.json())
curl -X POST https://freemail.maev.rocks/send/email_info \
-H "Content-Type: application/json" \
-d '{
"email_id": "12345",
"api_key": "your_api_key"
}'
// Using fetch
const getEmailInfo = async () => {
const response = await fetch('https://freemail.maev.rocks/send/email_info', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
email_id: '12345',
api_key: 'your_api_key'
})
});
const data = await response.json();
console.log(data);
};
getEmailInfo();
package main
import (
"bytes"
"encoding/json"
"fmt"
"net/http"
"io/ioutil"
)
func main() {
url := "https://freemail.maev.rocks/email_info"
data := map[string]string{
"email_id": "12345",
"api_key": "your_api_key",
}
jsonData, _ := json.Marshal(data)
resp, err := http.Post(url, "application/json", bytes.NewBuffer(jsonData))
if err != nil {
fmt.Println("Error:", err)
return
}
defer resp.Body.Close()
body, _ := ioutil.ReadAll(resp.Body)
fmt.Println("Response:", string(body))
}
use reqwest::Client;
use serde_json::json;
use tokio;
#[tokio::main]
async fn main() -> Result<(), reqwest::Error> {
let client = Client::new();
let url = "https://freemail.maev.rocks/email_info";
let payload = json!({
"email_id": "12345",
"api_key": "your_api_key"
});
let response = client
.post(url)
.header("Content-Type", "application/json")
.json(&payload)
.send()
.await?;
println!("Response: {:?}", response.text().await?);
Ok(())
}
Response
{
"status": "success",
"email": {
"id": "12345",
"sender_name": "Your Name",
"receiver_email": "recipient@example.com",
"email_subject": "Hello from Freemail API",
"message_content": "This is a test email sent using Freemail API.",
"email_type": "plain",
"is_template": false,
"footer": "Optional footer text",
"sent_at": "2025-05-21 14:45:00"
}
}
Error Responses
{
"status": "error",
"message": "Invalid API key"
}
{
"status": "error",
"message": "Missing email_id parameter"
}
{
"status": "error",
"message": "Email not found"
}
{
"status": "error",
"message": "Unauthorized access to this email"
}
Test Console
Test sending emails directly from your browser
A FREE email API for Developers
Send emails from your apps with just a few lines of code
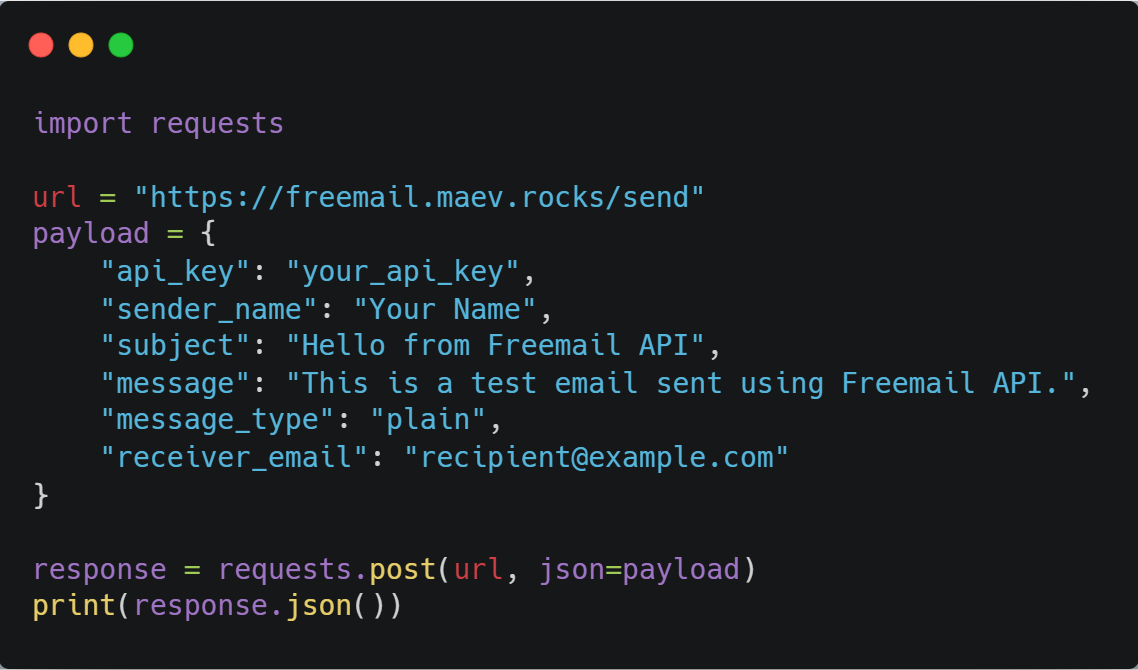
Simple Integration
Integrate with just a few lines of code using our RESTful API
Secure & Reliable
Built with security in mind, ensuring your emails are delivered safely
Real-time Analytics
Track delivery rates, opens, and engagement in real-time
Free Tier
Start with our generous free tier - no credit card required